{
"cells": [
{
"cell_type": "markdown",
"metadata": {},
"source": [
"# Fine-tune `dolly-v2-7b` with Ray Train, PyTorch Lightning and FSDP\n",
"\n",
"\n",
" \n",
"\n",
" "
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"In this example, we demonstrate how to use Ray Train to fine-tune a [`dolly-v2-7b`](https://huggingface.co/databricks/dolly-v2-7b) model. `dolly-v2-7b` is a 7 billion parameter causal language model created by Databricks, derived from EleutherAI’s [Pythia-6.9b](https://huggingface.co/EleutherAI/pythia-6.9b), and fine-tuned on a [~15K record instruction corpus](https://github.com/databrickslabs/dolly/tree/master/data).\n",
"\n",
"We load the pre-trained model from the HuggingFace model hub into a LightningModule and launch an FSDP fine-tuning job across 16 T4 GPUs with the help of {class}`Ray TorchTrainer `. It is also straightforward to fine-tune other similar large language models in a similar manner as shown in this example.\n",
"\n",
"Before starting this example, we highly recommend reading [Ray Train Key Concepts](train-key-concepts) and [Ray Data Quickstart](data_quickstart)."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Set up ray cluster \n",
"In this example, we are using a Ray cluster with a `g4dn.8xlarge` head node and 15 `g4dn.4xlarge` worker nodes. Each instance has one Tesla T4 GPU (16GiB Memory). \n",
"\n",
"We define a `runtime_env` to install the necessary Python libraries on each node. You can skip this step if you have already installed all the required packages in your workers' base image. We tested this example with `lightning==2.0.2` and `transformers==4.29.2`."
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {
"tags": []
},
"outputs": [],
"source": [
"import ray\n",
"\n",
"ray.init(\n",
" runtime_env={\n",
" \"pip\": [\n",
" \"datasets\",\n",
" \"evaluate\",\n",
" \"transformers>=4.26.0\",\n",
" \"torch>=1.12.0\",\n",
" \"lightning>=2.0\",\n",
" ]\n",
" }\n",
")"
]
},
{
"cell_type": "code",
"execution_count": 2,
"metadata": {
"tags": []
},
"outputs": [],
"source": [
"MODEL_NAME = \"databricks/dolly-v2-7b\""
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Prepare your data \n",
"We are using tiny_shakespeare for fine-tuning, which contains 40,000 lines of Shakespeare from a variety of Shakespeare's plays. Featured in Andrej Karpathy's blog post ['The Unreasonable Effectiveness of Recurrent Neural Networks'](http://karpathy.github.io/2015/05/21/rnn-effectiveness/). \n",
"\n",
"Dataset samples:\n",
"```\n",
"BAPTISTA:\n",
"I know him well: you are welcome for his sake.\n",
"\n",
"GREMIO:\n",
"Saving your tale, Petruchio, I pray,\n",
"Let us, that are poor petitioners, speak too:\n",
"Baccare! you are marvellous forward.\n",
"\n",
"PETRUCHIO:\n",
"O, pardon me, Signior Gremio; I would fain be doing.\n",
"```\n",
"\n",
"Here, we have adopted similar pre-processing logic from another demo: {doc}`GPT-J-6B Fine-Tuning with Ray Train and DeepSpeed <../deepspeed/gptj_deepspeed_fine_tuning>`."
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {
"tags": []
},
"outputs": [],
"source": [
"import ray\n",
"import pandas as pd\n",
"from datasets import load_dataset\n",
"from transformers import AutoTokenizer, AutoModelForCausalLM\n",
"\n",
"def split_text(batch: pd.DataFrame) -> pd.DataFrame:\n",
" text = list(batch[\"text\"])\n",
" flat_text = \"\".join(text)\n",
" split_text = [\n",
" x.strip()\n",
" for x in flat_text.split(\"\\n\")\n",
" if x.strip() and not x.strip()[-1] == \":\"\n",
" ]\n",
" return pd.DataFrame(split_text, columns=[\"text\"])\n",
"\n",
"\n",
"def tokenize(batch: pd.DataFrame) -> dict:\n",
" tokenizer = AutoTokenizer.from_pretrained(MODEL_NAME, padding_side=\"left\")\n",
" tokenizer.pad_token = tokenizer.eos_token\n",
" ret = tokenizer(\n",
" list(batch[\"text\"]),\n",
" truncation=True,\n",
" max_length=256,\n",
" padding=\"max_length\",\n",
" return_tensors=\"np\",\n",
" )\n",
" ret[\"labels\"] = ret[\"input_ids\"].copy()\n",
" return dict(ret)\n",
"\n",
"hf_dataset = load_dataset(\"tiny_shakespeare\")\n",
"train_ds = ray.data.from_huggingface(hf_dataset[\"train\"])"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We first split the original paragraphs into multiple sentences, then tokenize them. Here are some samples:"
]
},
{
"cell_type": "code",
"execution_count": 4,
"metadata": {
"tags": []
},
"outputs": [
{
"name": "stderr",
"output_type": "stream",
"text": [
"2023-08-30 11:03:12,182\tINFO dataset.py:2380 -- Tip: Use `take_batch()` instead of `take() / show()` to return records in pandas or numpy batch format.\n",
"2023-08-30 11:03:12,185\tINFO streaming_executor.py:93 -- Executing DAG InputDataBuffer[Input] -> TaskPoolMapOperator[MapBatches(split_text)] -> LimitOperator[limit=10]\n",
"2023-08-30 11:03:12,186\tINFO streaming_executor.py:94 -- Execution config: ExecutionOptions(resource_limits=ExecutionResources(cpu=None, gpu=None, object_store_memory=None), locality_with_output=False, preserve_order=False, actor_locality_enabled=True, verbose_progress=False)\n",
"2023-08-30 11:03:12,187\tINFO streaming_executor.py:96 -- Tip: For detailed progress reporting, run `ray.data.DataContext.get_current().execution_options.verbose_progress = True`\n"
]
},
{
"data": {
"application/vnd.jupyter.widget-view+json": {
"model_id": "e398e697cafb4b548fabc85020df5e87",
"version_major": 2,
"version_minor": 0
},
"text/plain": [
"Running 0: 0%| | 0/1 [00:00, ?it/s]"
]
},
"metadata": {},
"output_type": "display_data"
},
{
"data": {
"text/plain": [
"[{'text': 'Before we proceed any further, hear me speak.'},\n",
" {'text': 'Speak, speak.'},\n",
" {'text': 'You are all resolved rather to die than to famish?'},\n",
" {'text': 'Resolved. resolved.'},\n",
" {'text': 'First, you know Caius Marcius is chief enemy to the people.'},\n",
" {'text': \"We know't, we know't.\"},\n",
" {'text': \"Let us kill him, and we'll have corn at our own price.\"},\n",
" {'text': \"Is't a verdict?\"},\n",
" {'text': \"No more talking on't; let it be done: away, away!\"},\n",
" {'text': 'One word, good citizens.'}]"
]
},
"execution_count": 4,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"# First split the dataset into multiple sentences.\n",
"train_ds = train_ds.map_batches(split_text, batch_format=\"pandas\")\n",
"train_ds.take(10)"
]
},
{
"cell_type": "code",
"execution_count": 5,
"metadata": {},
"outputs": [],
"source": [
"# Then tokenize the dataset.\n",
"train_ds = train_ds.map_batches(tokenize, batch_format=\"pandas\")"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Define your lightning model\n",
"\n",
"In this example, we use the [dolly-v2-7b](https://huggingface.co/databricks/dolly-v2-7b) model for finetuning. It is an instruction-following large language model trained on the Databricks machine learning platform that is licensed for commercial use. We load the model weights from Huggingface Model Hub and encapsulate it into a `pl.LightningModule`.\n",
"\n",
":::{note}\n",
"Make sure you pass the FSDP wrapped model parameters `self.trainer.model.parameters()` into the optimizer, instead of `self.model.parameters()`. \n",
":::\n"
]
},
{
"cell_type": "code",
"execution_count": 6,
"metadata": {
"tags": []
},
"outputs": [],
"source": [
"import torch\n",
"import lightning.pytorch as pl\n",
"\n",
"class DollyV2Model(pl.LightningModule):\n",
" def __init__(self, lr=2e-5, eps=1e-8):\n",
" super().__init__()\n",
" self.save_hyperparameters()\n",
" self.lr = lr\n",
" self.eps = eps\n",
" self.model = AutoModelForCausalLM.from_pretrained(MODEL_NAME)\n",
"\n",
" def forward(self, batch):\n",
" outputs = self.model(\n",
" batch[\"input_ids\"], \n",
" attention_mask=batch[\"attention_mask\"], \n",
" labels=batch[\"labels\"]\n",
" )\n",
" return outputs.loss\n",
"\n",
" def training_step(self, batch, batch_idx):\n",
" loss = self.forward(batch)\n",
" self.log(\"train_loss\", loss, prog_bar=True, on_step=True)\n",
" return loss\n",
"\n",
" def configure_optimizers(self):\n",
" if self.global_rank == 0:\n",
" print(self.trainer.model)\n",
" return torch.optim.AdamW(self.trainer.model.parameters(), lr=self.lr, eps=self.eps)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Configure your FSDP strategy\n",
"As `dolly-v2-7b` is a relatively large model, it cannot be properly fit into a single commercial GPU. In this example, we use the FSDP strategy to shard model parameters across multiple workers. This allows us to avoid GPU out-of-memory issues and support a larger global batch size.\n",
"\n",
"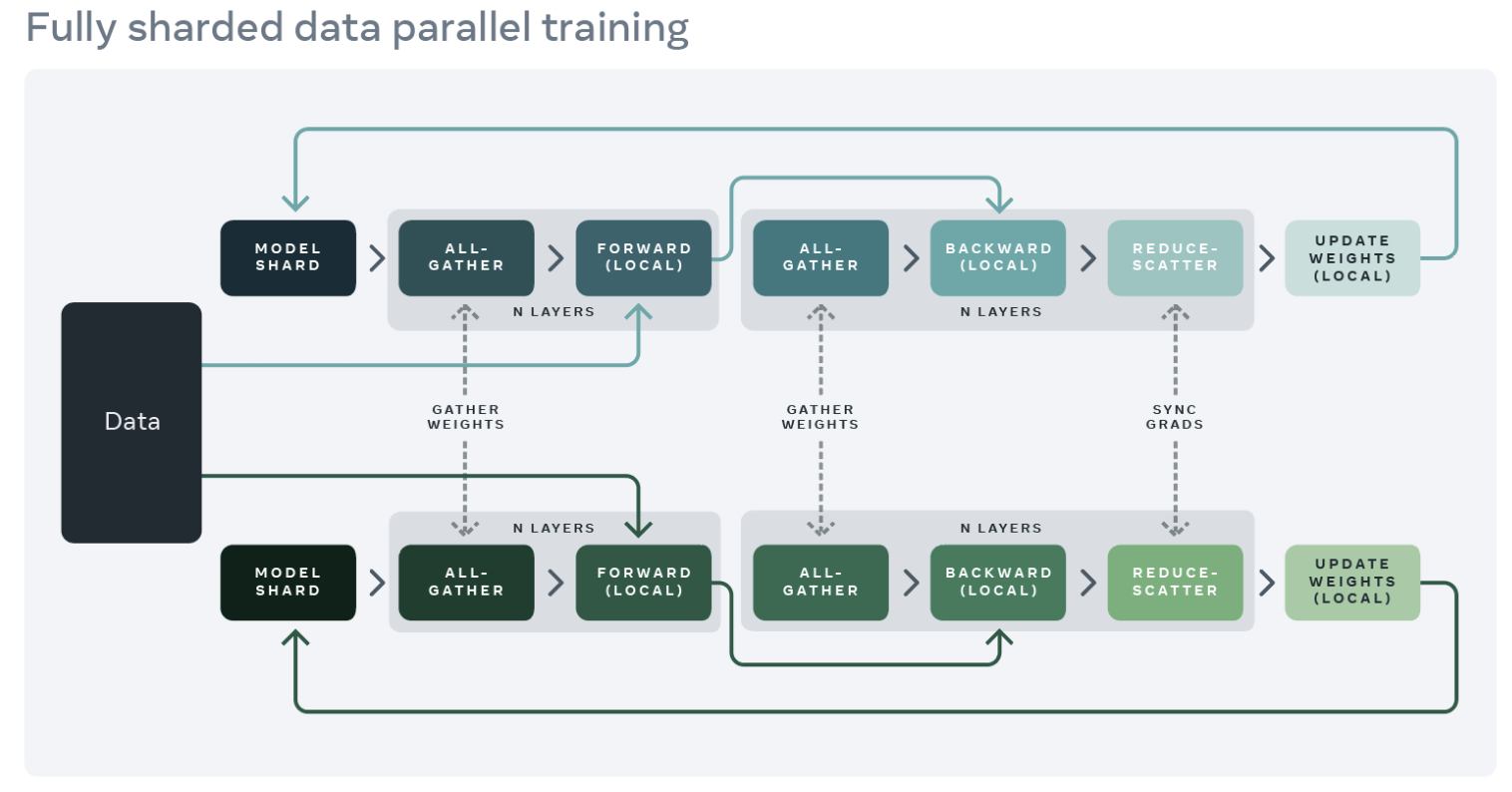\n",
"Image source: [Fully Sharded Data Parallel: faster AI training with fewer GPUs](https://engineering.fb.com/2021/07/15/open-source/fsdp/)\n",
"\n",
":::{note}\n",
"FSDP is a type of data parallelism that shards model parameters, optimizer states and gradients across DDP ranks. This was inspired by Xu et al. as well as the ZeRO Stage 3 from DeepSpeed. You may refer to these blogs for more information:\n",
"\n",
"- [Fully Sharded Data Parallel: faster AI training with fewer GPUs](https://engineering.fb.com/2021/07/15/open-source/fsdp/)\n",
"- [Getting Started with Fully Sharded Data Parallel(FSDP)](https://pytorch.org/tutorials/intermediate/FSDP_tutorial.html#:~:text=FSDP%20is%20a%20type%20of,sizes%20for%20our%20training%20job.)\n",
"- [PyTorch FSDP Tutorial](https://www.youtube.com/watch?v=8_k76AHu__s&list=PL_lsbAsL_o2BT6aerEKgIoufVD_fodnuT)\n",
":::\n",
"\n",
"To start training with Lightning's [FSDPStrategy](https://lightning.ai/docs/pytorch/stable/api/lightning.pytorch.strategies.FSDPStrategy.html#lightning.pytorch.strategies.FSDPStrategy), you only need to create a {class}`~ray.train.lightning.RayFSDPStrategy` with the same initialization arguments.\n"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {
"tags": []
},
"outputs": [],
"source": [
"import functools\n",
"import lightning.pytorch as pl \n",
"\n",
"from torch.distributed.fsdp.wrap import transformer_auto_wrap_policy\n",
"from torch.distributed.fsdp import ShardingStrategy, BackwardPrefetch\n",
"from transformers.models.gpt_neox.modeling_gpt_neox import GPTNeoXLayer\n",
"\n",
"from ray.train.lightning import RayFSDPStrategy\n",
"\n",
"\n",
"# Define the model sharding policy:\n",
"# Wrap every GPTNeoXLayer as its own FSDP instance\n",
"auto_wrap_policy = functools.partial(\n",
" transformer_auto_wrap_policy,\n",
" transformer_layer_cls = {GPTNeoXLayer}\n",
")\n",
"\n",
"fsdp_strategy = RayFSDPStrategy(\n",
" sharding_strategy=ShardingStrategy.FULL_SHARD,\n",
" backward_prefetch=BackwardPrefetch.BACKWARD_PRE,\n",
" forward_prefetch=True,\n",
" auto_wrap_policy=auto_wrap_policy,\n",
" limit_all_gathers=True,\n",
" activation_checkpointing=[GPTNeoXLayer],\n",
")"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
":::{tip}\n",
"\n",
"Some tips for FSDP configuration:\n",
"- `sharding_strategy`:\n",
" - `ShardingStrategy.NO_SHARD`: Parameters, gradients, and optimizer states are not sharded. Similar to DDP.\n",
" - `ShardingStrategy.SHARD_GRAD_OP`: Gradients and optimizer states are sharded during computation, and additionally, parameters are sharded outside computation. Similar to ZeRO stage-2.\n",
" - `ShardingStrategy.FULL_SHARD`: Parameters, gradients, and optimizer states are sharded. It has minimal GRAM usage among the 3 options. Similar to ZeRO stage-3.\n",
"- `auto_wrap_policy`:\n",
" - Model layers are often wrapped with FSDP in a layered fashion. This means that only the layers in a single FSDP instance are required to aggregate all parameters to a single device during forwarding or backward calculations.\n",
" - Use `transformer_auto_wrap_policy` to automatically wrap each Transformer Block into a single FSDP instance. \n",
"- `backward_prefetch` and `forward_prefetch`:\n",
" - Overlap the upcoming all-gather while executing the current forward/backward pass. It can improve throughput but may slightly increase peak memory usage.\n",
":::"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Fine-tune with Ray TorchTrainer\n",
"\n",
"Ray TorchTrainer allows you to scale your PyTorch Lightning training workload over multiple nodes. See {ref}`Configuring Scale and GPUs ` for more details."
]
},
{
"cell_type": "code",
"execution_count": 8,
"metadata": {},
"outputs": [],
"source": [
"num_workers = 16\n",
"batch_size_per_worker = 10"
]
},
{
"cell_type": "code",
"execution_count": 9,
"metadata": {
"tags": [
"remove-cell"
]
},
"outputs": [],
"source": [
"# To accelerate release tests\n",
"train_ds = train_ds.limit(num_workers * batch_size_per_worker * 10) # each worker has 10 batches"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Additionally, remember to define a Lightning callback that saves and reports checkpoints. Ray Train offers a simple implementation, {meth}`~ray.train.lightning.RayTrainReportCallback`, which persists your checkpoint and metrics in remote storage at the end of each training epoch. \n",
"\n",
"Note you can also implement your own report callback with customized logics, such as saving customized checkpoint files or reporting at a different frequency."
]
},
{
"cell_type": "code",
"execution_count": 10,
"metadata": {
"tags": [
"remove-cell"
]
},
"outputs": [
{
"name": "stderr",
"output_type": "stream",
"text": [
"2023-08-30 11:03:16,651\tINFO streaming_executor.py:93 -- Executing DAG InputDataBuffer[Input] -> TaskPoolMapOperator[MapBatches(split_text)->MapBatches(tokenize)]\n",
"2023-08-30 11:03:16,652\tINFO streaming_executor.py:94 -- Execution config: ExecutionOptions(resource_limits=ExecutionResources(cpu=None, gpu=None, object_store_memory=None), locality_with_output=False, preserve_order=False, actor_locality_enabled=True, verbose_progress=False)\n",
"2023-08-30 11:03:16,652\tINFO streaming_executor.py:96 -- Tip: For detailed progress reporting, run `ray.data.DataContext.get_current().execution_options.verbose_progress = True`\n"
]
},
{
"data": {
"application/vnd.jupyter.widget-view+json": {
"model_id": "a0e66d43f7d44da0a99483390e78e113",
"version_major": 2,
"version_minor": 0
},
"text/plain": [
"Running 0: 0%| | 0/1 [00:00, ?it/s]"
]
},
"metadata": {},
"output_type": "display_data"
},
{
"name": "stderr",
"output_type": "stream",
"text": [
"Downloading (…)okenizer_config.json: 100%|██████████| 450/450 [00:00<00:00, 66.9kB/s] \n",
"Downloading (…)/main/tokenizer.json: 0%| | 0.00/2.11M [00:00, ?B/s])\u001b[0m \n",
"Downloading (…)/main/tokenizer.json: 100%|██████████| 2.11M/2.11M [00:00<00:00, 14.8MB/s]\n",
"Downloading (…)cial_tokens_map.json: 100%|██████████| 228/228 [00:00<00:00, 143kB/s]m \n"
]
}
],
"source": [
"from lightning.pytorch.callbacks import TQDMProgressBar\n",
"\n",
"# Create a customized progress bar for Ray Data Iterable Dataset\n",
"class DollyV2ProgressBar(TQDMProgressBar):\n",
" def __init__(self, num_iters_per_epoch, *args, **kwargs):\n",
" super().__init__(*args, **kwargs)\n",
" self.num_iters_per_epoch = num_iters_per_epoch\n",
" \n",
" def on_train_epoch_start(self, trainer, *_):\n",
" super().on_train_epoch_start(trainer, *_)\n",
" self.train_progress_bar.reset(self.num_iters_per_epoch)\n",
"\n",
"num_iters_per_epoch = train_ds.count() // (num_workers * batch_size_per_worker)\n",
"prog_bar = DollyV2ProgressBar(num_iters_per_epoch)"
]
},
{
"cell_type": "code",
"execution_count": 11,
"metadata": {},
"outputs": [],
"source": [
"from ray.train import Checkpoint\n",
"from ray.train.lightning import RayLightningEnvironment, RayTrainReportCallback, prepare_trainer\n",
"\n",
"# Training function for each worker\n",
"def train_func(config):\n",
" lr = config[\"lr\"]\n",
" eps = config[\"eps\"]\n",
" strategy = config[\"strategy\"]\n",
" batch_size_per_worker = config[\"batch_size_per_worker\"]\n",
"\n",
" # Model\n",
" model = DollyV2Model(lr=lr, eps=eps)\n",
"\n",
" # Ray Data Ingestion\n",
" train_ds = ray.train.get_dataset_shard(\"train\")\n",
" train_dataloader = train_ds.iter_torch_batches(batch_size=batch_size_per_worker)\n",
"\n",
" # Lightning Trainer\n",
" trainer = pl.Trainer(\n",
" max_epochs=1, \n",
" devices=\"auto\",\n",
" accelerator=\"auto\", \n",
" precision=\"16-mixed\",\n",
" strategy=strategy,\n",
" plugins=[RayLightningEnvironment()],\n",
" callbacks=[RayTrainReportCallback()],\n",
" enable_checkpointing=False,\n",
" )\n",
"\n",
" trainer = prepare_trainer(trainer)\n",
"\n",
" trainer.fit(model, train_dataloaders=train_dataloader)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"```{note}\n",
"Since this example runs with multiple nodes, we need to persist checkpoints\n",
"and other outputs to some external storage for access after training has completed.\n",
"**You should set up cloud storage or NFS, then replace `storage_path` with your own cloud bucket URI or NFS path.**\n",
"\n",
"See the [storage guide](tune-storage-options) for more details.\n",
"```"
]
},
{
"cell_type": "code",
"execution_count": 12,
"metadata": {},
"outputs": [],
"source": [
"storage_path=\"s3://your-bucket-here\" # TODO: Set up cloud storage\n",
"# storage_path=\"/mnt/path/to/nfs\" # TODO: Alternatively, set up NFS"
]
},
{
"cell_type": "code",
"execution_count": 13,
"metadata": {
"tags": [
"remove-cell"
]
},
"outputs": [],
"source": [
"storage_path = \"/mnt/cluster_storage\""
]
},
{
"cell_type": "code",
"execution_count": 14,
"metadata": {
"tags": []
},
"outputs": [
{
"data": {
"text/html": [
"